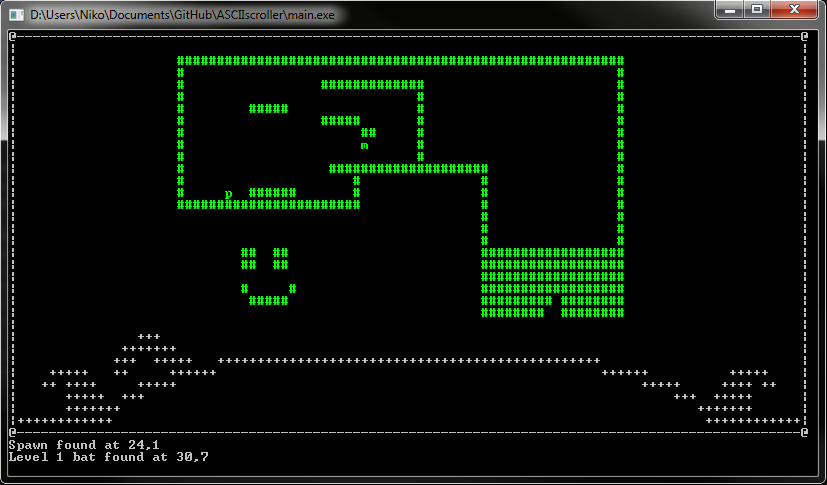
I’ve made significant progress today. I’ve learned (kind of) how to use pointers properly and effectively, in the sense that some of the things I did could not be done without them. I’ve also run into some of the odd things about C++, for instance that you can’t return an array from a function.
Graphics
Continuing where I left off yesterday, I ended up modifying the way that my graphics code worked for performance reasons. As you might remember, I was drawing each layer individuallyon to the screen. The windows console is not optimised for being able to do this, so by the time I had a functioning game I quickly realised that running at 5fps was simply not an option and that I was going to have to change the function that was taking the most time to do: drawing all the layers.
To solve this problem, I ended up creating a function called compress_layers(). This function takes all of the layers and puts them into one layer (in order) BEFORE the console actually prints them out. This way, every frame is only one write to the console, and all of the layer processing is done behind the scenes. This improved the performance remarkably, but still not to the level that I needed.
My next problem was a bit more difficult to be okay with changing (and I’m still not happy with it). My drawLayer() function was working very well up to this point, and it worked by running through every character in the layer and writing it individually to the console (in it’s designated position). This allowed me the freedom to color each character as I wanted to, which was great and gave the game a lot more character. Here’s what my *current code* looks like with the *old* drawLayer() function:
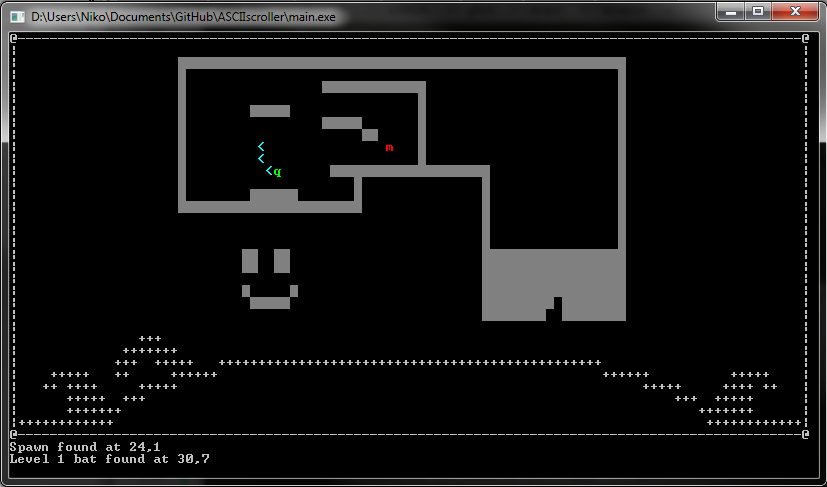
Pretty, right? Unfortunately going through each character was killing my framerate, so I was forced to write each line individually, meaning I can’t choose the individual colours. I’m still looking for a workaround for this, and I stumbled across http://www.tomshardware.com/forum/65918-13-technique-fast-win32-console-drawing which might be able to help me with that, but for now I’m unfortunately left with having a single printed colour :(.
Game Loop
Today, I also implemented a very basic game loop that runs physics 20 times per second, but lets the computer refresh the monitor as fast as it can. This isn’t ideal because it means my computer is working way harder than it needs to, but it’s functional which is enough for now.
Physics
Today I actually added physics to the game. An entity can either be flagged to be effected by physics or not. If it isn’t, then the physics function simply moves the object accordingly, but if it is it will also try to affect the Entity with gravity (making it fall). In the image above, “m” (a bat) is not affected by gravity, so it simply flies left and right on the screen, whereas the player (“p”) is affected by gravity, and therefore falls.
Input Handling
The player can now move left, right, jump, and shoot a projectile. In the image above, the player is facing left, shooting two projectiles, and is jumping in the air.
Reflection
Looking back at my code, I’m very happy with some of the way that my code is set up. The modules are almost entirely independent of eachother; all the entities are held in a vector, which is then modified by each game state, and drawn to the screen independently. Most of the functions and classes are independent of one another(modularization) which is something that I am constantly striving to improve in my games. My main function is very concise and manages to do very little work itself. The one thing that is still left in the Main() function that I’d ideally like separated is that the input handling is all located inside the main() loop: ideally I’d want it in it’s own header.
Tomorrow
Tomorrow is my last day to finish up this game. Tomorrow, I’d like to add:
- Hopefully I can figure out the console color issue: it’s a real bummer not to be able to make it look prettier.
- More enemies + AI
- An actual level system, saved progress (gold, powerups, etc.)
- Title screen
- Save games (even if it’s just to a text file)
- Loaded levels (from text files)
- Interaction between projectiles and enemies
I don’t expect to be able to do all of this, but I’ll do as much as I can!